Git
How to Create a New Git Branch: A Step-by-Step Guide
Branching is one of Git’s most powerful features, enabling developers to work on different versions of a project simultaneously. A branch in Git represents an independent line of development, making it perfect for implementing new features, fixing bugs, or experimenting with new ideas without impacting the main codebase.
In this blog, we’ll cover how to create a new branch in Git, along with some best practices to help you manage branches effectively.
1. Why Use Branches in Git?
Branches help isolate changes to specific features or fixes, making them invaluable for collaborative development. Using branches allows each team member to work on different tasks independently and seamlessly merge changes when ready. This workflow prevents conflicts, keeps the codebase clean, and makes it easier to roll back changes if needed.
2. Basic Git Branching Workflow
When working with Git, your project typically has a main branch (often called main
or master
). This branch is considered the stable version of the project. Creating new branches allows you to make changes without affecting this stable version. Here’s a typical workflow:
- Create a new branch for the task or feature.
- Make and commit changes on this new branch.
- Merge the branch into
main
once it’s ready.
3. How to Create a New Branch in Git
Creating a new branch is a straightforward process. Git provides commands to help you create and switch to new branches seamlessly.
Step 1: Open Your Terminal or Git Bash
Open your terminal (or Git Bash on Windows) and navigate to the local repository where you want to create the branch.
cd path/to/your/repo
Step 2: Check Your Current Branch
It’s a good practice to check which branch you’re currently on before creating a new branch. Use the following command:
git branch
This will list all the branches in your repository and highlight the branch you’re currently on with an asterisk (*).
Step 3: Create a New Branch
To create a new branch, use the following command:
git branch <branch-name>
Replace <branch-name>
with the name of your new branch. For example, to create a branch for a new feature called feature-login
, you would run:
git branch feature-login
This command creates a new branch based on the branch you’re currently on (usually main
), but it does not switch you to the new branch immediately.
Step 4: Switch to the New Branch
After creating a branch, switch to it with the following command:
git checkout <branch-name>
For example:
git checkout feature-login
Now, you’re on the feature-login
branch, and any changes you make will be saved there without affecting other branches.
Shortcut: Alternatively, you can create and switch to the new branch in one command by using -b
:
git checkout -b <branch-name>
Example:
git checkout -b feature-login
4. Verifying Your Branch
To verify that you’re on the correct branch, run:
git branch
The new branch should be listed, with an asterisk next to its name to indicate it’s the active branch.
5. Making and Committing Changes on Your New Branch
Once on the new branch, you can start making changes to your files. After making changes, you’ll want to commit them. Here’s the process:
- Add Changes to the Staging Area: Use
git add
to add your changes.
git add .
- Commit Your Changes: Use
git commit
to save your changes in the branch.
git commit -m "Add feature for user login"
These commands will commit your changes to the new branch (feature-login
), keeping the main
branch unaffected.
6. Pushing the New Branch to a Remote Repository
If you’re working in a team or have a remote repository, you’ll likely want to push your branch to a remote server (e.g., GitHub, GitLab).
Use the following command to push your branch:
git push -u origin <branch-name>
For example:
git push -u origin feature-login
The -u
flag sets up a tracking relationship between your local branch and the remote branch. This makes it easier to push updates in the future by simply running git push
.
7. Merging Your Branch into Main
Once you’ve completed your changes and are ready to incorporate them into the main codebase, you’ll need to merge the branch. Here’s a quick overview:
- Switch to the
main
branch:
git checkout main
- Pull the Latest Changes: Ensure your main branch is up to date.
git pull origin main
- Merge Your Feature Branch:
git merge feature-login
- Push the Updated Main Branch:
git push origin main
After merging, your changes from the feature branch will be integrated into the main branch.
8. Deleting the Branch (Optional)
If you no longer need the feature branch, it’s good practice to delete it to keep your branch list organized.
To delete a branch locally, use:
git branch -d <branch-name>
For example:
git branch -d feature-login
To delete a branch from the remote repository, use:
git push origin --delete <branch-name>
9. Branch Naming Best Practices
Using descriptive names for branches helps improve collaboration and project organization. Here are some naming conventions to consider:
- Feature Branches: Use
feature-
prefix (e.g.,feature-login
,feature-dashboard
). - Bug Fix Branches: Use
fix-
prefix (e.g.,fix-login-bug
,fix-header-layout
). - Hotfix Branches: Use
hotfix-
prefix for urgent fixes (e.g.,hotfix-security-patch
).
Following consistent naming conventions makes it easier for everyone on the team to understand the purpose of each branch.
10. Summary
Branching is a fundamental skill in Git that enhances collaboration, makes development faster, and improves code organization. To create a branch:
- Use
git branch <branch-name>
to create the branch. - Switch to it with
git checkout <branch-name>
. - Make changes and commit them to the branch.
- Push the branch to a remote repository if needed.
- Merge the branch into
main
when the changes are complete.
By following these steps and best practices, you can manage branches in Git effectively, keeping your project organized and your codebase clean.
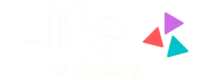