Q Sharp
Q Language – Type Casting: A Comprehensive Guide
In Q language, type casting (also known as type conversion) is the process of converting data from one type to another. Since Q is a strongly typed language, ensuring the correct data type is essential for efficient data manipulation and analysis in KDB+.
In this blog, we will explore:
✅ What is type casting in Q?
✅ Implicit vs. Explicit type casting
✅ How to convert data types in Q
✅ Practical examples
1. What Is Type Casting in Q?
Type casting in Q allows you to convert data between different types, such as:
- Numbers (integers, floats)
- Characters (symbols, strings)
- Temporal types (date, time, timestamp)
This is particularly useful when handling mixed datasets, performing mathematical operations, or preparing data for analysis.
2. Implicit vs. Explicit Type Casting
🔹 Implicit Type Casting (Automatic Conversion)
Q automatically converts data types when necessary. For example, performing arithmetic between an integer and a float results in a float.
Example of Implicit Type Casting:
q) 10 + 2.5
12.5
q) type 10 + 2.5
"f" / Float (real number)
✅ The integer (10
) is automatically converted to a float (10.0
) before addition.
🔹 Explicit Type Casting (Manual Conversion)
In explicit type casting, you manually change the data type using Q type-casting functions.
Example of Explicit Type Casting:
q) `int$12.5
12
q) type `int$12.5
"i" / Integer
✅ The float (12.5
) is explicitly converted to an integer (12
).
3. How to Convert Data Types in Q
Q provides casting operators ($
) and type functions to convert between different types. Below are some common conversions:
🔹 Convert String to Symbol
q) `symbol$"apple"
`apple
q) type `symbol$"apple"
"s" / Symbol
🔹 Convert Symbol to String
q) string `banana
"banana"
q) type string `banana
"c" / Character (string)
🔹 Convert Integer to Float
q) `float$42
42f
q) type `float$42
"f" / Float
🔹 Convert Float to Integer
q) `int$12.9
12
q) type `int$12.9
"i" / Integer
⚠️ Note: Conversion from float to integer removes the decimal part (truncation).
🔹 Convert Date to Timestamp
q) `timestamp$2024.02.06
2024.02.06D00:00:00.000000000
q) type `timestamp$2024.02.06
"p" / Timestamp
🔹 Convert Timestamp to Date
q) `date$2024.02.06D12:30:00.000000000
2024.02.06
q) type `date$2024.02.06D12:30:00.000000000
"d" / Date
🔹 Convert Boolean to Integer
q) `int$true
1
q) `int$false
0
✅ true
is converted to 1
, and false
is converted to 0
.
4. Handling Type Casting in Lists
You can apply type casting to entire lists in Q.
Example: Convert a List of Integers to Floats
q) `float$ 1 2 3
1f 2f 3f
q) type `float$ 1 2 3
"F" / List of floats
Example: Convert List of Strings to Symbols
q) `symbol$ ("apple"; "banana"; "grape")
`apple `banana `grape
q) type `symbol$ ("apple"; "banana"; "grape")
"S" / List of symbols
5. Why Type Casting Matters in Q?
✔ Ensures data consistency (e.g., converting strings to symbols for faster queries).
✔ Prevents type errors (e.g., using correct types in calculations).
✔ Optimizes performance (e.g., using integer types instead of floats when possible).
✔ Enhances compatibility when working with mixed data sources.
6. Conclusion
Type casting in Q is essential for handling data effectively. Whether you’re converting between numbers, symbols, strings, or time-based data, understanding how to use implicit and explicit type conversion improves both accuracy and performance in your KDB+ applications.
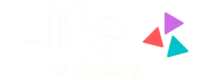