CSS
A Foolproof Guide to CSS How to Hide an Element
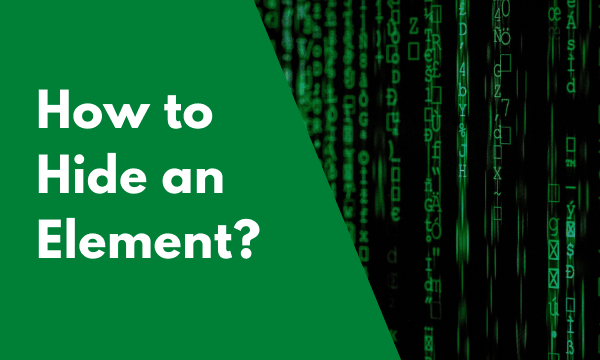
In this blog, you will learn How to hide an element using CSS with an easy to use property.
Contents
- 5 Scenarios to hide elements
- Practical Code to hide an element using CSS
- How to hide element but keep the space?
- How to hide element for mobile device?
- How to hide element after x seconds?
- How to hide element if empty?
- How to hide element for printing?
- How do i hide an element without display none?
- Conclusion
There comes a time when we have to hide some elements in the document in particular scenarios of web application.
Hiding the elements means the element is there in the document but it is not visible on the screen. There are multiple ways to hide an element & they serve the same purpose but have different effects on the document model of a web page.
Jquery or Javascript can be used to hide the elements on particular events on a web page. The scripting language will modify the CSS properties & hide the elements when the event occurs.
The elements can be shown or hidden based on the programming logic & it is easy to toggle the display of the elements.
5 Scenarios to hide elements
There are so many instances that demand to hide the elements on the screen based on certain events on the web page.
Below are just a few examples of using hide elements on client-side scripting.
- We may have come across some websites which display a pop up when you try to scroll the webpage. Pop up is hidden at the page load but appears after scrolling through the page.
- When there are lists of items that are displayed on the screen & a delete button is present to delete all the items, but in case there are no items then the delete button has no point to display so it is to be hidden.
- On a dashboard page, different widgets are displayed & there is a close button at top right corner of every widget. When clicked on the close button the widget box gets hidden.
- We might want to hide some elements on the web browser but they should be visible in mobile devices. Example is a button to open the website in an app.
- In a shopping card website, if the product has various colors then by clicking one color the product images are displayed for that color & other images are hidden at the same time.
Practical Code to hide an element using CSS
To hide an element, we use display property & apply it to the elements & this property determines the display of the elements. When we want to hide an element then we need to set the none value to this property.
You can either use inline style & apply it directly to an element or through internal CSS file.
When we desire to unhide an element then we can use the same display property & set the value to block or inline-block.
Display property can also be changed with javascript or jquery & the display of the element can be toggled on the various events on the page.
There are various types of elements that are displayed on the web page like p, headings, span, table, div, etc. & all of these can be hidden with the display property.
Following is the easy to use Code :-
CSS :-
.hideMe { display: none; } div { display: inline-block; border: solid 1px red; width: 200px; height: 100px; text-align: center; }
HTML :-
<div> I am a div. </div> <div class="hideMe"> I am hidden. </div> <div> I am a div after hidden div. </div>
Result :-
In the above code, 3 div elements are defined that have some text within every div.
The middle div is applied to the class .hideMe & the CSS for this class is helping to hide this element with the use of display : none property.
As you can see in the Result image, the middle div is hidden without occupying any space. There is no trace left of the middle div but the element stays in DOM.
How to hide element but keep the space?
When we choose to hide the element then two things can happen regarding the space of the element. One thing is space will be erased & another thing is space will be available even after the element is hidden.
visibility property can be used to hide the elements. It hides the element but the space of the element still remains visible on the web page.
This property does the same job of hiding the element with a behavior that the size of the element will be occupied on the page. All rest properties of the element like borders, colors, text styles will be hidden with the element but the width & height specified for the element will remain.
In some instances, hiding elements on the page can disturb the structure of the web page & design can move completely. Visibility property can come handy in such a situation.
To bring back the hidden element, visibility property can be set to visible & it will make the element appear again on the screen.
Let’s see the Code below :-
CSS :-
.hideMe { visibility: hidden; } div { display: inline-block; border: solid 1px red; width: 200px; height: 100px; text-align: center; }
HTML :-
<div> I am a div. </div> <div class="hideMe"> I am hidden. </div> <div> I am a div after hidden div. </div>
Result :-
In the HTML code block, 3 div’s are displayed with some content. The middle div is applied the class hideMe & the class will be applied as defined in CSS.
In the CSS section, we have used the visibility property to hide the element. Visibility is set to hidden so the element will be hidden & it will keep the space for this element on the web page.
In the Result image you can notice that there is a space between 2 divs & that space is the width & height of the middle div that is hidden.
How to hide element for mobile device?
Mobile devices have a small width & the website takes a different look when viewed in the smaller devices.
Website design takes a new dimension when it is viewed on mobile devices. This creates a need to change the elements on the web page to display differently in browsers & mobiles.
Bigger elements on the web browser sometimes need to be kept hidden in the mobiles. So the question arises how to hide element for mobile device.
The answer to this question is CSS media queries.
Media queries allows us to define dimensions & apply the styles to the elements based on the size dimensions of the device.
CSS :-
<style> div { border: solid 1px red; padding: 20px; } @media screen and (max-width: 768px) { .mobileDiv { display: none!important; } } </style>
HTML :-
<div> I will be displayed both in Browser & Mobiles. </div> <div class="mobileDiv"> I will be automatically hidden in Mobile devices. </div>
Result in Browser :-
Result in Mobile :-
In the above code sample, the @media screen does the trick.
We have specified max-width to 768px, this means that the CSS defined within the media screen will be automatically applied when device width is less than or equal to 768px.
We have applied display : none for the class mobileDiv & the div will only be hidden in devices with or less than 768px width.
In the devices with greater than 768px width will display the div because display : none is only applied for less than 768px width.
How to hide element after x seconds?
In certain scenarios of web application, we need to erase some objects on the screen after x seconds. Sometimes the content is temporary & it has to be taken away from the user’s view after some seconds.
There are multiple ways to hide the element after x seconds. We are trying to provide the simplest solution that can be applied instantly.
We have to use the javascript code to hide the element & you can note that no jquery file is needed for this solution.
The element will be faded away after the specified seconds & it will not return back. If someone wants to return it back then he can but for this particular point we will make it disappear forever.
Disappearing element looks small but it creates a good impression in the user’s mind. It makes the page responsive & not static. Page becomes alive & does not look dead because page is responding to users action on the web page.
We will now see the practical code for hiding the element after x seconds.
CSS :-
<style> .hideMe { opacity: 0; } .hideMe_With_DisplayNone { display: none; } </style>
HTML :-
<div id="one">I will disappear after 5 seconds.</div>
Script :-
<script> setTimeout(function () { document.getElementById('one').className = 'hideMe'; }, 5000); </script>
In the above code, we have placed a div within HTML & then after 5 seconds it is programmed to disappear.
By the combination of javascript function setTimeout & CSS properties, the div element gets faded away from the screen.
Two ways are demonstrated in the above code to hide the element, first is by making the opacity to 0 & second is by using display none property.
In the above script section, setTimeOut function accepts 2 parameters, first is the function that is code that is defined & second parameter is milliseconds after which first parameter function will run.
In the function, we are using the code to apply class to the div by its id. As the class is applied to the div the opacity will be set as 0.
To use the display none, you can change the className to hideMe_With_DisplayNone.
How to hide element if empty?
There might be some elements in the html document that are empty. Empty means that element does not contain anything & there is a way to remove these elements using CSS.
We can actually hide these empty elements without knowing the id or class of the elements. If there are 20 div elements on the screen & 4 are empty then we do not need to know which exact 4 are empty for hiding purposes.
The removal of the empty elements will erase the unnecessary space that was occupied by them & the design of the web page will improve.
The element will be completely removed from the screen & it will not leave any trace in the screen.
The empty elements in the html document can be the byproduct of the application logic. The logic can be server side or client side that can sometimes result in empty elements due to the no content from the logic used.
Here’s the code :-
CSS :-
<style> div { border: solid 1px red; margin: 10px; padding: 10px; } div:empty { display: none } </style>
HTML :-
<div>I am not empty.</div> <div>I am also not empty.</div> <div>I am also not empty but next div is empty.</div> <div></div> <div>I am also not empty but previous div is empty.</div>
Result :-
Result without using empty :-
The placeholder :empty is used to identify the elements in the document that are empty. The CSS section of code selects the empty div’s & then hides them by using display none.
As you can see in the HTML section above that 5 div elements are created & the 4th div is empty.
In the normal scenario the 4th div will display with space occupied on screen as shown in the Result without using empty section.
With the use of :empty placeholder the 4th empty div will be hidden from the document.
How to hide element for printing?
If we do not want to print something this is not supposed to be printed then we have the choice to skip that element for printing in the browsers.
In the long content in the web page we can hide something that is irrelevant at the time of printing because printing means your content will be printed on a physical paper that will be distributed further.
The content will be visible on the screen with eyes, only at the time of printing the content you choose will be automatically skipped on the printing page.
The web developer will determine & use the CSS for hiding particular content while printing & it will not be in users hand.
From a user’s point of view, he will have no idea what is happening & why some part of content is not printing.
Let’s see the code below :-
CSS :-
<style> @media print { .do_not_print { display: none; } } </style>
HTML :-
<div class="do_not_print"> I will not be printed for sure. </div>
In the CSS block of code, we have used @media print & the styles given to the elements within this section will be automatically applied at the time of printing.
To hide the element, we have created a class with name do_not_print & applied display none.
In the HTML block of code, the class do_not_print is applied to the div element so this element will be hidden while printing on the browser.
How do i hide an element without display none?
There are multiple ways to hide an element from the screen. And every way has some kind of drawbacks that create a need to try a different solution.
Some CSS properties do not work on all the devices & most probably with older versions.
Below are some ways other than display none that can also be used for hiding element using CSS.
Move the element off the screen by applying positioning to absolute
In this trick, we apply a random bigger size value to the left property. The position of the element has also to be made absolute.
This will take the element to the way left in the screen & it will not be visible.
CSS :-
<style> .hideMe { position: absolute; left: -99999px; } </style>
Changing the opacity
With the help of opacity CSS property, we can hide the element from the eyes of the user by turning its opacity to 0.
The element will disappear from the screen like a ghost. It will be there but will not be visible to eyes.
CSS :-
<style> .hideMe { opacity: 0; } </style>
Applying negative margins
One more trick to hide elements is to set the margin with large negative numbers.
Margins also work with positive as well as negative numbers. The below defined class will throw the element off the screen & it will not be visible on the web page.
CSS :-
<style> .hideMe { margin-left: -999999px; } </style>
Conclusion
As you can see now that hiding an element isn’t a big deal. Applying simple CSS properties to the html elements does the job & hides the desired element instantly.
Same CSS properties can be toggled with the help of scripting language to show & hide the elements.
In certain scenarios, when the page loads we hide an element initially & then after some seconds we might need to display that element like the Pop up boxes to subscribe to our website or any other information in the pop up.
Showing & hiding elements comes very much handy when developing a web application.
Now you can go ahead & try the coding so that you can experience the same practically.